|
发表于 2007-9-26 00:46:19
|
显示全部楼层
|阅读模式
来自 中国–广西–柳州–城中区
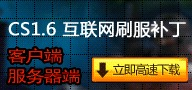 /***************************************************************************************
Colored Admin Chat - (c) 2007 by Exolent
Made by Exolent http://forums.alliedmods.net/showthread.php?t=60009
Where the idea came from: http://forums.alliedmods.net/showthread.php?t=57993
Description:
This plugin allows admin with the specified admin level to have colored text
as all the text in their messagemode and messagemode2 chat messages. Also.
"/command" messages and "@", "@@", and "@@@" messages will not be shown but
they still will work.
Cvars:
amx_cac_enabled <0|1> (default: 1)
amx_adminlisten_voice <0|1> (default: 1)
amx_adminlisten_voice <0|1> (default: 1)
*NOTE* This was ONLY tested in CS 1.6. Use in other mods at own risk.
This plugin's source and compiled files can be found @:
http://forums.alliedmods.net/showthread.php?t=60009
***************************************************************************************
Changelog:
Version 1.0
- Initial release
Version 1.1
- Added admin level for green chat
- Merged 4 format() functions into one
Version 2.0
- Simple code cleanup
- Merged AdminListen with fakemeta and not engine
--- AdminListen Link: http://forums.alliedmods.net/showthread.php?p=107768
Version 3.0
- Multiplied color possibilites
- Rearranged messages
- Fixed a few bugs
- Renamed plugin
Version 3.1
- Cleanup code
- Fixed cvar chat issue
Version 3.2
- Made only 1 check for @ | @@ | @@@ say commands
- Made voice follow sv_alltalk when not using voice listening
- Added cvar to turn on/off plugin
- Removed green name with green chat
- Optimized has_color_chat() and get_color_chat() when using get_user_flags()
- Removed SPEAK_MUTED for its uselessness
Version 4.0
- Rewrote code
- Fixed a few bugs
**************************************************************************************/
// COLOR CHAT LEVELS
// --------------------------------------------------------------------------------------
#define ADMIN_NORMAL ADMIN_LEVEL_A // level for normal chat with green name only
#define ADMIN_RED ADMIN_LEVEL_B // level for red chat
#define ADMIN_BLUE ADMIN_LEVEL_C // level for blue chat
#define ADMIN_SILVER ADMIN_LEVEL_D // level for silver chat
// --------------------------------------------------------------------------------------
// ADMIN LISTEN LEVELS
// --------------------------------------------------------------------------------------
#define LISTEN_CHAT ADMIN_KICK // level to see all chat (adminlisten style)
#define LISTEN_VOICE ADMIN_IMMUNITY // level to hear all voices (adminlisten style)
// --------------------------------------------------------------------------------------
// DO NOT EDIT BEYOND THIS LINE UNLESS YOU KNOW WHAT YOU ARE DOING
// --------------------------------------------------------------------------------------
#include <amxmodx>
#include <amxmisc>
#include <fakemeta>
#define SPEAK_NORMAL (1<<0)
//#define SPEAK_MUTED (1<<1)
#define SPEAK_ALL (1<<2)
#define SPEAK_LISTENALL (1<<3)
new pCvar_enabled;
new pCvar_voice;
new pCvar_chat;
new pCvar_alltalk;
new giMaxPlayers;
new giMsgSayText;
new giMsgTeamInfo;
new giSpeakFlags[33];
new const giColorLevels[] =
{
ADMIN_ALL,
ADMIN_NORMAL,
ADMIN_RED,
ADMIN_BLUE,
ADMIN_SILVER
};
new const gszColorCode[][] =
{
"",
"^x01",
"^x03",
"^x03",
"^x03"
};
new const gszColorTeams[][] =
{
"",
"",
"TERRORIST",
"CT",
"SPECTATOR"
};
new const gszTeamNames[][] =
{
"Spectator",
"Terrorist",
"Counter-Terrorist",
"Spectator"
};
public plugin_init()
{
register_plugin("Colored Admin Chat", "4.0", "Exolent");
register_clcmd("say", "handle_say");
//register_forward(FM_Voice_SetClientListening, "fm_SetVoice");
pCvar_enabled = register_cvar("amx_cac_enabled", "1");
pCvar_alltalk = get_cvar_pointer("sv_alltalk");
giMaxPlayers = get_maxplayers();
giMsgSayText = get_user_msgid("SayText");
giMsgTeamInfo = get_user_msgid("TeamInfo");
register_forward(FM_Voice_SetClientListening, "fm_SetVoice")
}
public handle_say(id)
{
if(!get_pcvar_num(pCvar_enabled))
return PLUGIN_CONTINUE;
static szMessage[192];
read_args(szMessage, 191);
remove_quotes(szMessage);
if(!is_valid_message(szMessage))
return PLUGIN_CONTINUE;
static bool:bAdmin;
bAdmin = has_color_chat(id);
static iChatColor, iAlive, i;
iChatColor = get_chat_color(id);
iAlive = is_user_alive(id);
static szName[32], szTeam[32], szTeam2[32];
get_user_name(id, szName, 31);
get_user_team(id, szTeam, 31);
format(szMessage, 191, "%s%s%s ^x01: %s %s",\
iAlive ? "" : "^x01*DEAD*",\
bAdmin ? "^x04" : "^x01",\
szName,\
bAdmin ? gszColorCode[iChatColor] : "",\
szMessage);
for(i = 1; i <= giMaxPlayers; i++)
{
if(!is_user_connected(i))
continue;
if(iAlive == is_user_alive(i)
|| (get_pcvar_num(pCvar_chat)) && (get_user_flags(i) & LISTEN_CHAT))
{
get_user_team(i, szTeam2, 31);
handle_message(i, szTeam, szTeam2, iChatColor, szMessage);
}
}
return PLUGIN_HANDLED;
}
public handle_teamsay(id)
{
if(!get_pcvar_num(pCvar_enabled))
return PLUGIN_CONTINUE;
static szMessage[192];
read_args(szMessage, 191);
remove_quotes(szMessage);
if(!is_valid_message(szMessage))
return PLUGIN_CONTINUE;
static bool:bAdmin;
bAdmin = has_color_chat(id);
static iChatColor, iAlive, iTeam, i;
iChatColor = get_chat_color(id);
iAlive = is_user_alive(id);
iTeam = get_user_team(id);
static szName[32], szTeam[32], szTeam2[32];
get_user_name(id, szName, 31);
get_user_team(id, szTeam, 31);
format(szMessage, 191, "^x01%s(%s)%s %s^x01 : %s %s",\
iAlive ? "" : "*DEAD*",\
gszTeamNames[iTeam],\
bAdmin ? "^x04" : "^x03",\
szName,\
bAdmin ? gszColorCode[iChatColor] : "",\
szMessage);
for(i = 1; i <= giMaxPlayers; i++)
{
if(!is_user_connected(i))
continue;
get_user_team(i, szTeam2, 31);
if(!equali(szTeam, szTeam2) && (get_pcvar_num(pCvar_chat) != 1))
continue;
if(iAlive == is_user_alive(i)
|| get_user_flags(i) & LISTEN_CHAT)
{
handle_message(id, szTeam, szTeam2, iChatColor, szMessage);
}
}
return PLUGIN_HANDLED;
}
public fm_SetVoice(iReceiver, iSender, bool:bListen)
{
reset_speak(iReceiver);
reset_speak(iSender);
if((giSpeakFlags[iSender] & SPEAK_ALL) != 0)
{
set_speak(iReceiver, iSender, true);
return FMRES_SUPERCEDE;
}
if((giSpeakFlags[iReceiver] & SPEAK_LISTENALL) != 0)
{
set_speak(iReceiver, iSender, true);
return FMRES_SUPERCEDE;
}
forward_return(FMV_CELL, bListen);
return FMRES_IGNORED;
}
bool:is_valid_message(const szMessage[])
{
if(szMessage[0] == '/'
|| szMessage[0] == '@'
|| !strlen(szMessage))
{
return false;
}
return true;
}
bool:has_color_chat(const id)
{
static iFlags, i;
iFlags = get_user_flags(id);
for(i = 1; i < sizeof giColorLevels; i++)
{
if(iFlags & giColorLevels)
return true;
}
return false;
}
get_chat_color(const id)
{
static iFlags, i;
iFlags = get_user_flags(id);
for(i = 1; i < sizeof giColorLevels; i++)
{
if(iFlags & giColorLevels)
return i;
}
return 0;
}
handle_message(const id, /*const*/ szTeam[], /*const*/ szTeam2[], const iChatColor, /*const*/ szMessage[])
{
if(equali(gszColorCode[iChatColor], "^x03") && !equali(gszColorTeams[iChatColor], szTeam2))
{
show_TeamInfo_msg(id, gszColorTeams[iChatColor]);
show_SayText_msg(id, szMessage);
show_TeamInfo_msg(id, szTeam2);
}
else if(!equali(szTeam, szTeam2) && !iChatColor)
{
show_TeamInfo_msg(id, szTeam);
show_SayText_msg(id, szMessage);
show_TeamInfo_msg(id, szTeam2);
}
else
{
show_SayText_msg(id, szMessage);
}
return 1;
}
show_TeamInfo_msg(const id, /*const*/ szTeam[])
{
message_begin(MSG_ONE, giMsgTeamInfo, _, id);
write_byte(id);
write_string(szTeam);
message_end();
}
show_SayText_msg(const id, /*const*/ szMessage[])
{
message_begin(MSG_ONE, giMsgSayText, _, id);
write_byte(id);
write_string(szMessage);
message_end();
}
reset_speak(const id)
{
if(get_pcvar_num(pCvar_alltalk) == 1)
{
giSpeakFlags[id] = SPEAK_ALL;
}
else if((get_pcvar_num(pCvar_voice) == 1) && (get_user_flags(id) & LISTEN_VOICE))
{
giSpeakFlags[id] = SPEAK_LISTENALL;
}
else
{
giSpeakFlags[id] = SPEAK_NORMAL;
}
}
set_speak(const iReceiver, const iSender, const bool:bListen)
{
engfunc(EngFunc_SetClientListening, iReceiver, iSender, bListen)
forward_return(FMV_CELL, bListen);
} 这是个说话不同颜色的插件源码,我现在想把里面的权限去掉改为按排名说话颜色不同,功能只要say就可以,调试了二个小时无果,麻烦你了,十分感谢。:burn: |
|